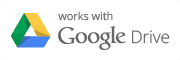
PHPでGoogle Drive Apiを使用する
最近だとクラウドにデータを入れて保存等を行うことがあります。
それはプログラムも一緒です。バッチ等で必要なデータをクラウドに保存することがあります。
今回はGoogle Drive ApiをPHPで使用する方法のご紹介。
認証の方法とファイル一覧の取得、ファイルの追加。
※現在2014/06/09の最新状態のGoogle Api php clientを使用しています。
アップデート等がありますとサンプル通りに動かない可能性があります。
目次
Google APIs Console の Drive API を ON にする
Google Api ConsoleにアクセスをしてAPIs & auth -> APIsでAPIのリストを表示。Google DriveのAPIを探して有効にしてください。

Google Drive Api の接続情報の作成
有効にしたGoogle Drive Apiから設定のページに移動して、API Accessを選択。【Create an Oauth…】のボタンを押下してください。
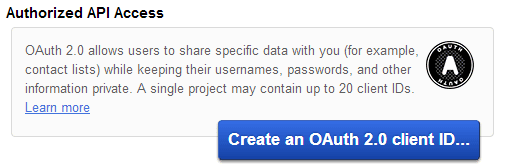
設定を進めていてください。
設定完了後に、APIで使用するIDが出力されます。

Google Api php clientの取得
Google API php Clientからダウンロード、解答を行ってください。私が書いたサンプルだと解凍後のsrcフォルダの中のGoogleフォルダを抜き出して使っています。
Google Drive Api使い方
Google Drive Apiの使い方の説明認証
Google Driveの認証を行う。ソースに書いてあるクライントID、シークレットID、リダイレクトURLは上記で設定したものを使用してください。
// ライブラリ読み込み require_once('./Google/Client.php'); // Google Drive require_once('./Google/Service/Drive.php'); // セッションスタート session_start(); $client = new Google_Client(); // クライアントID $client->setClientId('クライアントID'); // クライアントSecret ID $client->setClientSecret('クライアントSecret ID'); // リダイレクトURL $client->setRedirectUri('リダイレクトURL'); $service = new Google_Service_Drive($client); // 許可されてリダイレクトされると URL に code が付加されている // code があったら受け取って、認証する if (isset($_GET['code'])) { // 認証 $client->authenticate($_GET['code']); $_SESSION['token'] = $client->getAccessToken(); // リダイレクト GETパラメータを見えなくするため(しなくてもOK) header('Location: http://'.$_SERVER['HTTP_HOST'].$_SERVER['PHP_SELF']); exit; } // セッションからアクセストークンを取得 if (isset($_SESSION['token'])) { // トークンセット $client->setAccessToken($_SESSION['token']); } // トークンがセットされていたら if ($client->getAccessToken()) { try { echo "Google Drive Api 連携完了!"; } catch (Google_Exception $e) { echo $e->getMessage(); } } else { // 認証用URL取得 $client->setScopes(Google_Service_Drive::DRIVE); $authUrl = $client->createAuthUrl(); echo '<a href="'.$authUrl.'">アプリケーションのアクセスを許可してください。</a>'; }
ファイル一覧の取得
Google Driveのファイル一覧の取得方法。※認証済みが前提です。
try { // ライブラリ読み込み require_once('./Google/Client.php'); // Google Drive require_once('./Google/Service/Drive.php'); // 認証 session_start(); $client = new Google_Client(); $client->setAccessToken($_SESSION['token']); $service = new Google_Service_Drive($client); // 親ディレクトリ // root でマイドライブ, root 以外は名前ではなく ID を指定 $parents = 'root'; if (isset($_GET['parents'])) { $parents = htmlspecialchars($_GET['parents'], ENT_QUOTES); } // 次ページに移動する場合に渡すトークン $pageToken = null; if (isset($_GET['pageToken'])) { $pageToken = $_GET['pageToken']; } $parameters = array('q' => "'{$parents}' in parents", 'maxResults' => 20); if ($pageToken) { $parameters['pageToken'] =$pageToken; } // ファイルリスト取得, Google_Service_Drive_FileList のオブジェクトが返ってくる $files = $service->files->listFiles($parameters); // ファイルの一覧データ $results = $files->getItems(); // 次ページのトークン取得, ない場合は NULL $pageToken = $files->getNextPageToken(); // 結果表示 foreach ($results as $result) { // フォルダだったらリンクに if ($result->mimeType === 'application/vnd.google-apps.folder') { echo '<a href="http://'.$_SERVER['HTTP_HOST'].$_SERVER['PHP_SELF'].'?parents='.urlencode($result->id).'">フォルダ : '.$result->title.'</a><br />'; } else { echo "ファイル : {$result->title}<br />"; } } // pageToken があったら次ページヘのリンク表示 if ($pageToken) { echo '<a href="http://'.$_SERVER['HTTP_HOST'].$_SERVER['PHP_SELF'].'?parents='.urlencode($parents).'&pageToken='.urlencode($pageToken).'">次ページ</a>'; } } catch (Google_Exception $e) { echo $e->getMessage(); }
Google Driveにファイルを追加する
Google Driveにファイルを追加するサンプルです。※認証済みが前提です。
<?php // ライブラリ読み込み require_once('./Google/Client.php'); // Google Drive require_once('./Google/Service/Drive.php'); // 認証 session_start(); $client = new Google_Client(); $client->setAccessToken($_SESSION['token']); $service = new Google_Service_Drive($client); // 追加したいファイルオブジェクトを作成 $file = new Google_Service_Drive_DriveFile(); $file->setTitle('test.txt'); $file->setDescription('テストファイル'); $file->setMimeType('text/plain'); // Google Driveに登録するファイル $filename = 'test.txt'; $chunkSizeBytes = 1 * 1024 * 1024; // 親としたいフォルダの ID $parentId = 'root'; // 親オブジェクト $parent = new Google_Service_Drive_ParentReference(); $parent->setId($parentId); // ファイルに親をセット $file->setParents(array($parent)); // ファイルを送信してHTTP通信を開く $client->setDefer(true); $request = $service->files->insert($file); // アップするファイルオブジェクトを生成 $media = new Google_Http_MediaFileUpload( $client, $request, 'text/plain', null, true, $chunkSizeBytes ); $media->setFileSize(filesize($filename)); // ファイルを送信する $status = false; $handle = fopen($filename, "rb"); while (!$status && !feof($handle)) { $chunk = fread($handle, $chunkSizeBytes); $status = $media->nextChunk($chunk); } $result = false; if($status != false) { $result = $status; } fclose($handle); $client->setDefer(false);
参考
参考にしたサイトです。[PHP] Google Drive API を使って Google Drive のファイル一覧取得、ファイルの追加を行う
Quickstart: Run a Drive App in PHP
Google APIs Client Library for PHP
«前の記事:Google Chromeで日本国外からのアクセスになってしまうライセンスの種類と要点:次の記事»