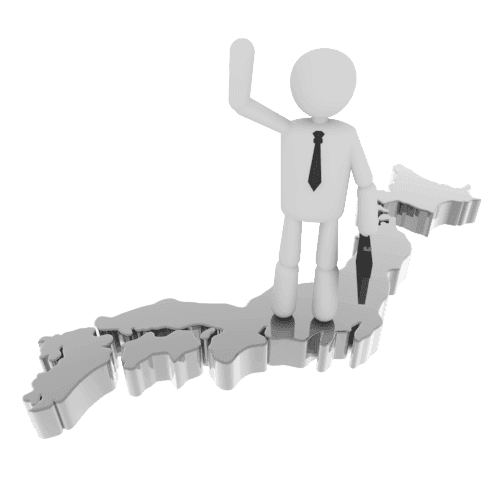
Google Maps APIで住所から緯度・経度を取得する方法
Google Maps API緯度・経度を使って地図にマーカー等を表示します。
ただ住所はわかっても緯度・経度何て普通はわかりません。
なので住所から緯度・経度を取得します。
住所・地名:
上記サンプルのコード
<!-- Google Maps APIの読み込み --> <script type='text/javascript' src='http://www.google.com/jsapi'></script> <script type='text/javascript'>google.load('jquery', '1.3.2');</script> <script type='text/javascript' src='http://maps.google.com/maps/api/js?sensor=false&language=ja' charset='UTF-8'></script> <script> // mapオブジェクトの初期化 map = null; // 初期設定 jQuery(document).ready(function () { initialize(); }); // マップ初期化 function initialize(){ var mapdiv = document.getElementById('map_canvas'); var geocoder = new google.maps.Geocoder(); var options = { // ズーム倍率 zoom : 8, // 地図中央の緯度・経度(新宿駅) center : new google.maps.LatLng(35.68978889880994, 139.70049233458258), // 地図タイプ mapTypeId : google.maps.MapTypeId.ROADMAP }; map = new google.maps.Map(mapdiv, options); } // 住所から緯度・経度取得 function getLatLng(place) { // マップを初期化 initialize(); // ジオコーダのコンストラクタ var geocoder = new google.maps.Geocoder(); // geocodeリクエストを実行。 // 第1引数はGeocoderRequest // 住所をaddressプロパティに入れる。 // 第2引数はコールバック関数。 geocoder.geocode({ address: place }, function(results, status) { if (status == google.maps.GeocoderStatus.OK) { // 結果の表示範囲。結果が1つとは限らないので、LatLngBoundsで用意。 var bounds = new google.maps.LatLngBounds(); for (var i in results) { if (results[i].geometry) { // 緯度経度を取得 var latlng = results[i].geometry.location; // 検索結果地が含まれるように範囲を拡大 bounds.extend(latlng); // マーカー var marker = new google.maps.Marker({ position: latlng, map: map }); // 情報ウィンドウを出力 var infowindow = new google.maps.InfoWindow({ content: results[i].formatted_address + "<br>(Lat, Lng) = " + latlng.toString() }); infowindow.open(map, marker); // ポップアップがクローズされた場合、マーカーを押されたらポップアップが開くイベントを付与 google.maps.event.addListener(infowindow , "closeclick", function() { google.maps.event.addListenerOnce(marker , "click", function(event) { infowindow.open(map, marker); }); }); } } // 範囲を移動 map.fitBounds(bounds); } else if (status == google.maps.GeocoderStatus.ERROR) { alert("Google サーバーへの接続に問題が発生しました。"); } else if (status == google.maps.GeocoderStatus.INVALID_REQUEST) { alert("このリクエストは無効でした。"); } else if (status == google.maps.GeocoderStatus.OVER_QUERY_LIMIT) { alert("ウェブページがリクエストの制限回数を超えました。"); } else if (status == google.maps.GeocoderStatus.REQUEST_DENIED) { alert("ウェブページで PlacesService の使用が許可されていません。"); } else if (status == google.maps.GeocoderStatus.UNKNOWN_ERROR) { alert("サーバー エラーのため PlacesService リクエストを処理できませんでした。\r\nもう一度実行すると、リクエストが正常に処理される可能性があります。"); } else if (status == google.maps.GeocoderStatus.ZERO_RESULTS) { alert("このリクエストに対する結果が見つかりませんでした。"); } else { alert("その他、不明"); } }); } </script> 住所・地名:<input type="text" value="桜木町" size="30" id="address"/> <input type="button" value="緯度・経度取得" onclick="getLatLng(document.getElementById('address').value)" /> <div class='map' id='map_canvas'></div>これで入力された住所から緯度・経度を求めてマーカーをつけたり移動が可能になります。
ただ、こちらには使用制限があり1秒間で10アクセス程しか出来ません。
それ以上、早くするとgoogle.maps.GeocoderStatus.OVER_QUERY_LIMITが返ってきます。
なので短時間に多量のアクセスは気をつけてください。
«前の記事:HTML5 バイブレーション APIMysql 開発で役立つSQL:次の記事»